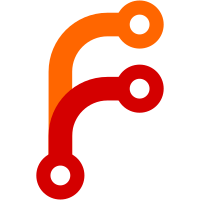
Co-authored-by: Flarp <flurpdadurp@gmail.com> Reviewed-on: benji/nswp#1 Co-authored-by: him <edorta@uncc.edu> Co-committed-by: him <edorta@uncc.edu>
37 lines
1,000 B
Java
37 lines
1,000 B
Java
package net.benjidial.nswp.commands;
|
|
|
|
import net.benjidial.nswp.Database;
|
|
import net.benjidial.nswp.Waypoint;
|
|
|
|
import org.bukkit.entity.Player;
|
|
|
|
import java.sql.SQLException;
|
|
|
|
public class RenameWaypoint extends WaypointCommand {
|
|
public CompletionType getCompletionType() {
|
|
return CompletionType.Waypoint;
|
|
}
|
|
|
|
@Override
|
|
public boolean body(Player player, String[] args) throws SQLException {
|
|
if (args.length != 2) return false;
|
|
|
|
if (Database.lookupWaypoint(player, args[1]) != null) {
|
|
player.sendMessage("Waypoint with that name already exists!");
|
|
return true;
|
|
}
|
|
|
|
Waypoint waypoint = Database.lookupWaypoint(player, args[0]);
|
|
|
|
if (waypoint == null) {
|
|
player.sendMessage("No waypoint with that name.");
|
|
} else {
|
|
Database.deleteWaypoint(player, args[0]);
|
|
waypoint.name = args[1];
|
|
Database.addWaypoint(player, waypoint);
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
}
|